Magento 2: API запрос метод PUT
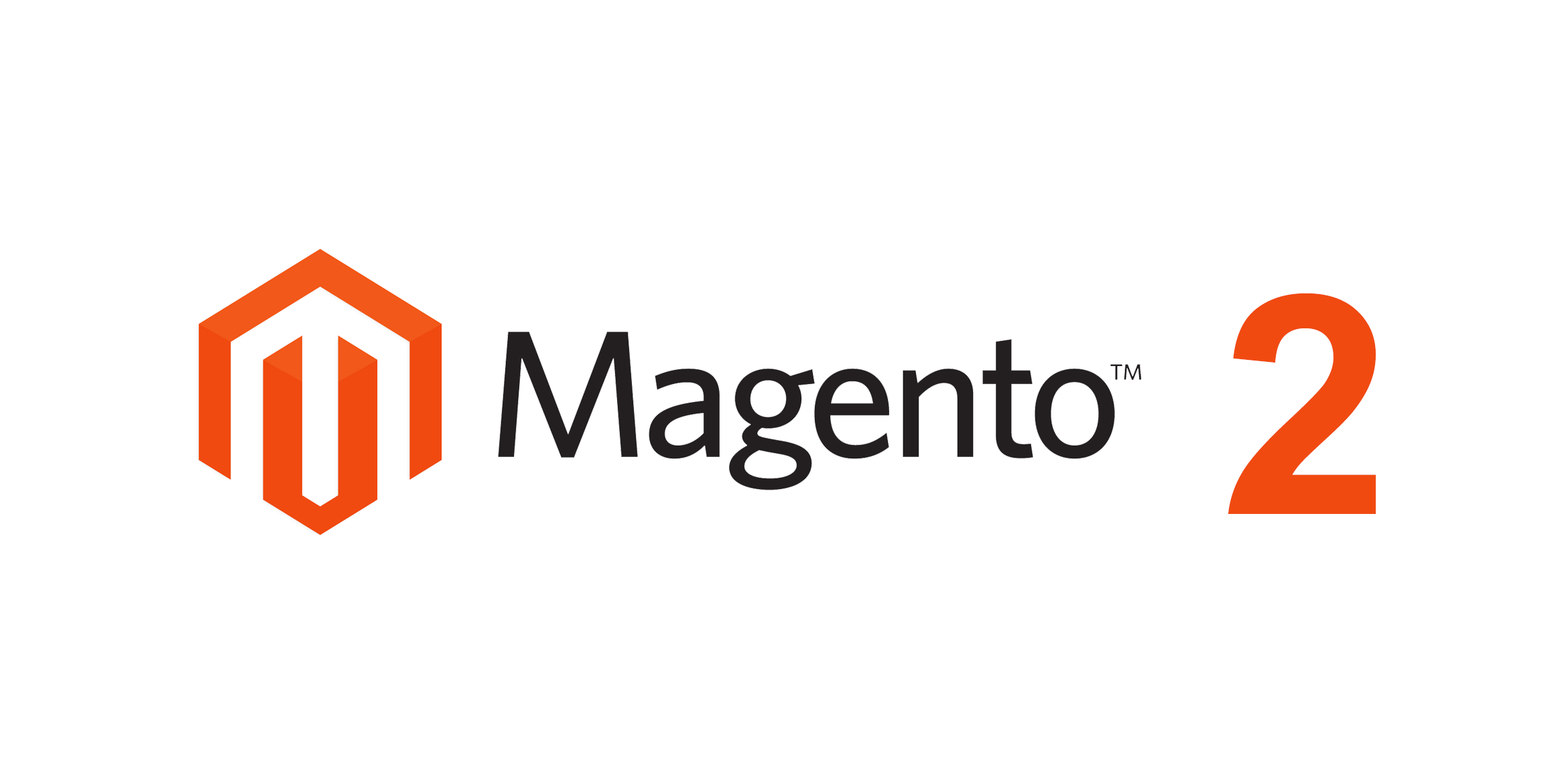
Пример получения данных в результате выполнения API запроса в контроллере Magento 2.
declare(strict_types=1); namespace ITStorm\ApiTest\Controller\Adminhtml\Send; use Magento\Backend\App\Action; use Magento\Framework\App\ResponseInterface; use Magento\Framework\Controller\ResultFactory; use Magento\Backend\App\Action\Context; use Magento\Framework\Controller\ResultInterface; use Magento\Framework\HTTP\ZendClient; use Psr\Log\LoggerInterface; use Magento\Framework\HTTP\ZendClientFactory; use Zend_Http_Response; class ApiTesting extends Action { public const API_URL = 'https://www.your-api.com'; /** * @var LoggerInterface */ private LoggerInterface $logger; /** * @var ZendClientFactory */ private ZendClientFactory $httpClientFactory; /** * ApiTesting constructor. * @param Context $context * @param LoggerInterface $logger * @param ZendClientFactory $httpClientFactory */ public function __construct( Context $context, LoggerInterface $logger, ZendClientFactory $httpClientFactory ) { $this->logger = $logger; $this->httpClientFactory = $httpClientFactory; parent::__construct($context); } /** * Получаем данные API запросом * * @return ResponseInterface|ResultInterface */ public function execute() { $data[ 'prodSomeId' => 12345; 'brand' => 'Sony'; ]; try { $apiResponse = $this->apiConnect(self::API_URL, $data, ZendClient::PUT); $apiData = $this->json->unserialize($apiResponse->getBody()); } catch (\Exception $e) { $this->logger->warning('ITStorm_ApiTest: ' . $e->getMessage()); } // Do something ... return $response; } /** * Perform API request and return result * * @param string $url * @param array $data * @return Zend_Http_Response * @throws \Zend_Http_Client_Exception */ public function apiConnect(string $url, array $data = [], string $method = ZendClient::GET): Zend_Http_Response { $client = $this->getHttpClient(); $client->setUri($url); $client->setHeaders([ 'Content-Type' => 'application/json' ]); if ($data) { $client->setRawData(json_encode($data), 'application/json'); $client->setMethod($method); } $response = $client->request(); $this->checkResponse($response); return $response; } private function getHttpClient(): ZendClient { $client = $this->httpClientFactory->create(); $client->setConfig([ 'maxredirects' => 1, 'timeout' => 30000 ]); return $client; } /** * Check response * * @param Zend_Http_Response $response * @throws \Exception * @return void */ private function checkResponse(Zend_Http_Response $response): void { if ($response->getStatus() !== 200) { throw new ApiErrorException(__('API error. Response code %1', $response->getStatus())); } } }