Magento 2: Выполнение запросов через API
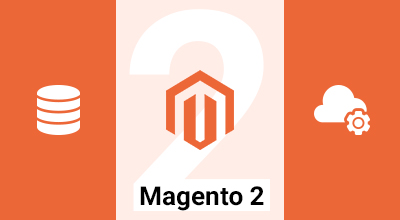
Использование Magento\Framework\HTTP для работы с API
Библиотека Magento\Framework\HTTP предоставляет удобный способ выполнения HTTP-запросов, включая методы PUT и другие, которые не поддерживаются стандартным cURL в Magento.Пример реализации:
use Magento\Framework\HTTP\ZendClientFactory; private $httpClientFactory; /** * Выполнение API-запроса и возврат результата * * @param string $url * @param array $data * @return Zend_Http_Response * @throws \Zend_Http_Client_Exception */ public function apiConnect(string $url, array $data = []): Zend_Http_Response { $client = $this->getHttpClient(); $client->setUri($url); $client->setHeaders([ 'Content-Type' => 'application/json' ]); if ($data) { $client->setRawData(json_encode($data), 'application/json'); $client->setMethod($client::POST); } $response = $client->request(); $this->checkResponse($response); return $response; } /** * Возвращает HTTP-клиент Zend * * @return ZendClient * @throws \Zend_Http_Client_Exception */ private function getHttpClient(): ZendClient { $client = $this->httpClientFactory->create(); $client->setConfig([ 'maxredirects' => 1, 'timeout' => 30000 ]); return $client; } /** * Проверка ответа API * * @param Zend_Http_Response $response * @throws \Exception */ private function checkResponse(Zend_Http_Response $response): void { if ($response->getStatus() !== 200) { throw new ApiErrorException(__('API error. Response code %1', $response->getStatus())); } }
Кастомный класс исключений
class ApiErrorException extends \Magento\Framework\Exception\LocalizedException { const PHRASE = 'Api error.'; public function __construct( \Magento\Framework\Phrase $phrase = null, \Exception $cause = null, $code = 0 ) { if ($phrase === null) { $phrase = new \Magento\Framework\Phrase(__(self::PHRASE)); } parent::__construct($phrase, $cause, $code); } }